Web components were on my rader as a native built-in feature that I was interested in using. But after using it, I have some thoughts on the technology.
y tho
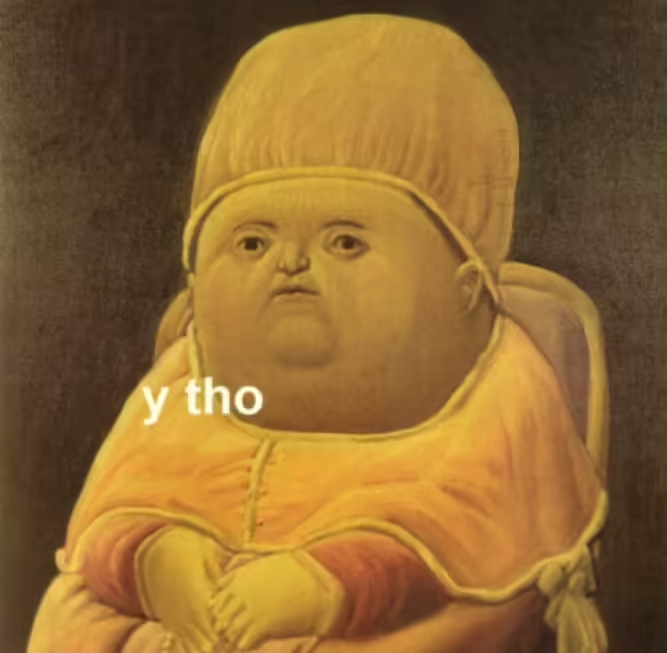
Well, lets start with my…
Experience
Honestly, the experience with Web Components (Atleast for me) wasn’t that bad. I was using it to add progressive enhancements / small interactivity in my site’s guestbook (Check it out at /guestbook). However, I was not using it the way most people would think.
Here is what I did:
<guestbook-comments class="mt-10 gap-4 flex flex-col">
<!-- child slot replaced with data fetched -->
<slot />
</guestbook-comments>
<script>
import type { GuestBookEntry } from "../utils/guestbook";
class GuestbookComments extends HTMLElement {
public async addEntry(newEntry: GuestBookEntry) {
// ...
}
}
</script>
You can find the full source file on GitHub.
Web components are meant to be like framework components (Like in React for example), where a component itself does everything in a shadow-root. However, a problem arises this way.
Web components don’t support SSR, and they also lead to worse SEO. Because they need to be defined with customElements.define()
which is only
available in the browser window
object. This means using shadow root as a namespace for creating elements will not render the elements if
JavaScript is disabled. They are client-only, and this is a deal breaker for me. These are meant to be small interactive bits, essentially an addEventListener()
call, not a fully “SPA-Like” component.
What I was doing with web components could be just used as regular onsubmit
&& onclick
handlers. The only advantage with using Web Components for
usual event listeners, was writing code where you have custom methods defined on an element.
<script>
class AddComment extends HTMLElement {
public async addComment() {
// Types are funny
const guestbookComments: (Element & { addData(newEntry: GuestBookEntry): Promise<void> }) | null = document.querySelector("guestbook-comments");
// Its the same method that I had defined before in the WC
if (guestbookComments) await guestbookComments.addEntry(stuff);
// ^^^^^^^
}
}
</script>
Do I think Web Components are good though?
Mostly negative.
I just think they can be better. The main issue I have with them is their API & SSR/SEO Limitations. I don’t get the trend of making native features ridiculously difficult to work with. Web Components don’t need to have a API that makes it difficult to work with them. Everytime a new feature comes out on the web, its either too low-level (like WCs) or too limited. There should be a balance between the 2 tradeoffs. For small interactivity websites? Meh, they can work. For larger website however? Absolutely not.
Conclusion
Although everyone likes to trash on Web Components, I think they are pretty alright, but only in rare scenarios. (I’ll admit that even I too have said crap about it before) They are a convenient feature for adding “interactivity” with vanilla JavaScript. Their utility is strengthened further when it comes to frameworks like Astro with the Islands architecture, however they still fail to show their pros.